mirror of
https://github.com/ldc-developers/ldc.git
synced 2025-05-13 06:28:52 +03:00
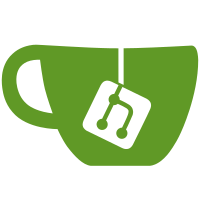
* Fixed a minor linking order mishap * * Added an command line option -annotate * * Fixed some problems with running optimizations * * Added std.stdio and dependencies to lphobos (still not 100% working, but compiles and links) * * Fixed problems with passing aggregate types to variadic functions * * Added initial code towards full GC support, currently based on malloc and friends, not all the runtime calls the GC yet for memory * * Fixed problems with resolving nested function context pointers for some heavily nested cases * * Redid function argument passing + other minor code cleanups, still lots to do on this end... *
21 lines
511 B
D
21 lines
511 B
D
|
|
/*
|
|
* Placed in public domain.
|
|
* Written by Hauke Duden and Walter Bright
|
|
*/
|
|
|
|
/* This is for use with variable argument lists with extern(D) linkage. */
|
|
|
|
/* Modified for LLVMDC (LLVM D Compiler) by Tomas Lindquist Olsen, 2007 */
|
|
|
|
module std.stdarg;
|
|
|
|
alias void* va_list;
|
|
|
|
T va_arg(T)(ref va_list vp)
|
|
{
|
|
size_t size = T.sizeof > size_t.sizeof ? size_t.sizeof : T.sizeof;
|
|
va_list vptmp = cast(va_list)((cast(size_t)vp + size - 1) & ~(size - 1));
|
|
vp = vptmp + T.sizeof;
|
|
return *cast(T*)vptmp;
|
|
}
|