mirror of
https://github.com/ldc-developers/ldc.git
synced 2025-05-09 04:15:58 +03:00
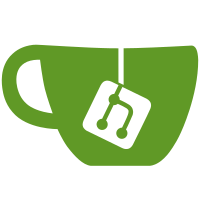
Also adds the CMake infrastructure to compile and link the D source files. The build is partially broken: - A few files in Phobos and druntime do not build - MSVC build is broken because of unresolved symbols involving reals
106 lines
2.8 KiB
C++
106 lines
2.8 KiB
C++
|
|
/* Compiler implementation of the D programming language
|
|
* Copyright (c) 1999-2014 by Digital Mars
|
|
* All Rights Reserved
|
|
* written by Walter Bright
|
|
* http://www.digitalmars.com
|
|
* Distributed under the Boost Software License, Version 1.0.
|
|
* http://www.boost.org/LICENSE_1_0.txt
|
|
* https://github.com/D-Programming-Language/dmd/blob/master/src/mars.h
|
|
*/
|
|
|
|
#ifndef DMD_MARS_H
|
|
#define DMD_MARS_H
|
|
|
|
#ifdef __DMC__
|
|
#pragma once
|
|
#endif
|
|
|
|
/*
|
|
It is very important to use version control macros correctly - the
|
|
idea is that host and target are independent. If these are done
|
|
correctly, cross compilers can be built.
|
|
The host compiler and host operating system are also different,
|
|
and are predefined by the host compiler. The ones used in
|
|
dmd are:
|
|
|
|
Macros defined by the compiler, not the code:
|
|
|
|
Compiler:
|
|
__DMC__ Digital Mars compiler
|
|
_MSC_VER Microsoft compiler
|
|
__GNUC__ Gnu compiler
|
|
__clang__ Clang compiler
|
|
|
|
Host operating system:
|
|
_WIN32 Microsoft NT, Windows 95, Windows 98, Win32s,
|
|
Windows 2000, Win XP, Vista
|
|
_WIN64 Windows for AMD64
|
|
__linux__ Linux
|
|
__APPLE__ Mac OSX
|
|
__FreeBSD__ FreeBSD
|
|
__OpenBSD__ OpenBSD
|
|
__sun Solaris, OpenSolaris, SunOS, OpenIndiana, etc
|
|
|
|
For the target systems, there are the target operating system and
|
|
the target object file format:
|
|
|
|
Target operating system:
|
|
TARGET_WINDOS Covers 32 bit windows and 64 bit windows
|
|
TARGET_LINUX Covers 32 and 64 bit linux
|
|
TARGET_OSX Covers 32 and 64 bit Mac OSX
|
|
TARGET_FREEBSD Covers 32 and 64 bit FreeBSD
|
|
TARGET_OPENBSD Covers 32 and 64 bit OpenBSD
|
|
TARGET_SOLARIS Covers 32 and 64 bit Solaris
|
|
|
|
It is expected that the compiler for each platform will be able
|
|
to generate 32 and 64 bit code from the same compiler binary.
|
|
|
|
There are currently no macros for byte endianness order.
|
|
*/
|
|
|
|
|
|
#include <stdio.h>
|
|
#include <stdint.h>
|
|
#include <stdarg.h>
|
|
#if IN_LLVM
|
|
#include <stddef.h>
|
|
#include <stdarg.h>
|
|
#endif
|
|
|
|
#ifdef __DMC__
|
|
#ifdef DEBUG
|
|
#undef assert
|
|
#define assert(e) (static_cast<void>((e) || (printf("assert %s(%d) %s\n", __FILE__, __LINE__, #e), halt())))
|
|
#endif
|
|
#endif
|
|
|
|
void unittests();
|
|
|
|
struct OutBuffer;
|
|
|
|
#include "globals.h"
|
|
|
|
#include "longdouble.h"
|
|
|
|
#include "complex_t.h"
|
|
|
|
#include "errors.h"
|
|
|
|
class Dsymbol;
|
|
class Library;
|
|
struct File;
|
|
void obj_start(char *srcfile);
|
|
void obj_end(Library *library, File *objfile);
|
|
void obj_append(Dsymbol *s);
|
|
void obj_write_deferred(Library *library);
|
|
|
|
void readFile(Loc loc, File *f);
|
|
void writeFile(Loc loc, File *f);
|
|
void ensurePathToNameExists(Loc loc, const char *name);
|
|
|
|
const char *importHint(const char *s);
|
|
/// Little helper function for writting out deps.
|
|
void escapePath(OutBuffer *buf, const char *fname);
|
|
|
|
#endif /* DMD_MARS_H */
|