mirror of
https://github.com/ldc-developers/ldc.git
synced 2025-05-03 16:41:06 +03:00
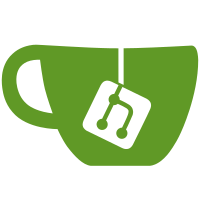
Note: For a backward compatible interface, use the new bin/ldmd script. It supports all old options while passing on anything it doesn't recognize. Some changes caused by this: * -debug and -version are now -d-debug and -d-version due to a conflict with standard LLVM options. * All "flag" options now allow an optional =true/=1/=false/=0 suffix. * Some "hidden debug switches" starting with "--" were renamed because LLVM doesn't care about the number of dashes, so they were conflicting with other options (such as -c). The new versions start with "-hidden-debug-" instead of "--" * --help works, but has a non-zero exit code. This breaks some Tango scripts which use it to test for compiler existence. See tango.patch. Some changes not (directly) caused by this; * (-enable/-disable)-FOO options are now available for pre- and postconditions. * -march is used instead of -m (like other LLVM programs), but -m is an alias for it. * -defaultlib, -debuglib, -d-debug and -d-version allow comma-separated values. The effect should be identical to specifying the same option multiple times. I decided against allowing these for some other options because paths might contain commas on some systems. * -fPIC is removed in favor of the standard LLVM option -relocation-model=pic Bug: * If -run is specified as the last argument in DFLAGS, no error is generated. (Not very serious IMHO)
85 lines
1.7 KiB
C++
85 lines
1.7 KiB
C++
#include <cassert>
|
|
#include <cstdarg>
|
|
#include <cstdio>
|
|
#include <cstdlib>
|
|
#include <iostream>
|
|
#include <fstream>
|
|
#include <string>
|
|
|
|
#include "mars.h"
|
|
|
|
#include "llvm/Support/CommandLine.h"
|
|
#include "gen/logger.h"
|
|
|
|
namespace Logger
|
|
{
|
|
static std::string indent_str;
|
|
static std::ofstream null_out("/dev/null");
|
|
|
|
llvm::cl::opt<bool> _enabled("vv",
|
|
llvm::cl::desc("Very verbose"),
|
|
llvm::cl::ZeroOrMore);
|
|
|
|
void indent()
|
|
{
|
|
if (_enabled) {
|
|
indent_str += "* ";
|
|
}
|
|
}
|
|
void undent()
|
|
{
|
|
if (_enabled) {
|
|
assert(!indent_str.empty());
|
|
indent_str.resize(indent_str.size()-2);
|
|
}
|
|
}
|
|
std::ostream& cout()
|
|
{
|
|
if (_enabled)
|
|
return std::cout << indent_str;
|
|
else
|
|
return null_out;
|
|
}
|
|
void println(const char* fmt,...)
|
|
{
|
|
if (_enabled) {
|
|
printf("%s", indent_str.c_str());
|
|
va_list va;
|
|
va_start(va,fmt);
|
|
vprintf(fmt,va);
|
|
va_end(va);
|
|
printf("\n");
|
|
}
|
|
}
|
|
void print(const char* fmt,...)
|
|
{
|
|
if (_enabled) {
|
|
printf("%s", indent_str.c_str());
|
|
va_list va;
|
|
va_start(va,fmt);
|
|
vprintf(fmt,va);
|
|
va_end(va);
|
|
}
|
|
}
|
|
void enable()
|
|
{
|
|
_enabled = true;
|
|
}
|
|
void disable()
|
|
{
|
|
_enabled = false;
|
|
}
|
|
bool enabled()
|
|
{
|
|
return _enabled;
|
|
}
|
|
void attention(const Loc& loc, const char* fmt,...)
|
|
{
|
|
printf("Warning: %s: ", loc.toChars());
|
|
va_list va;
|
|
va_start(va,fmt);
|
|
vprintf(fmt,va);
|
|
va_end(va);
|
|
printf("\n");
|
|
}
|
|
}
|