mirror of
https://github.com/ldc-developers/ldc.git
synced 2025-05-04 00:55:49 +03:00
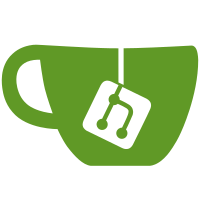
DMD has the obscure functionality to install functions starting with _STI_ as global ctors and funtions starting with _STD_ as global dtors. IMHO a pragma is a better way to specify the behaviour. This commit adds pragma(LDC_global_crt_ctor) and pragma(LDC_global_crt_dtor). If the pragma is specified on a function or static method then an entry is made in the corresponding list. E.g. in monitor_.d: extern (C) { #pragma(LDC_global_crt_ctor) void _STI_monitor_staticctor() { // ... } } This works on Linux without problems. On Windows with MS C Runtime ctors work always but dtors are invoked only if linked against the static C runtime. Dtors on Windows require at least LLVM 3.2.
54 lines
1.3 KiB
C++
54 lines
1.3 KiB
C++
//===-- gen/pragma.h - LDC-specific pragma handling -------------*- C++ -*-===//
|
||
//
|
||
// LDC – the LLVM D compiler
|
||
//
|
||
// This file is distributed under the BSD-style LDC license. See the LICENSE
|
||
// file for details.
|
||
//
|
||
//===----------------------------------------------------------------------===//
|
||
//
|
||
// Code for handling the LDC-specific pragmas.
|
||
//
|
||
//===----------------------------------------------------------------------===//
|
||
|
||
#ifndef PRAGMA_H
|
||
#define PRAGMA_H
|
||
|
||
#include <string>
|
||
|
||
struct PragmaDeclaration;
|
||
struct Dsymbol;
|
||
struct Scope;
|
||
|
||
enum Pragma
|
||
{
|
||
LLVMnone, // Not an LDC pragma.
|
||
LLVMignore, // Pragma has already been processed in DtoGetPragma, ignore.
|
||
LLVMintrinsic,
|
||
LLVMglobal_crt_ctor,
|
||
LLVMglobal_crt_dtor,
|
||
LLVMno_typeinfo,
|
||
LLVMno_moduleinfo,
|
||
LLVMalloca,
|
||
LLVMva_start,
|
||
LLVMva_copy,
|
||
LLVMva_end,
|
||
LLVMva_arg,
|
||
LLVMinline_asm,
|
||
LLVMinline_ir,
|
||
LLVMfence,
|
||
LLVMatomic_store,
|
||
LLVMatomic_load,
|
||
LLVMatomic_cmp_xchg,
|
||
LLVMatomic_rmw,
|
||
LLVMbitop_bt,
|
||
LLVMbitop_btc,
|
||
LLVMbitop_btr,
|
||
LLVMbitop_bts
|
||
};
|
||
|
||
Pragma DtoGetPragma(Scope *sc, PragmaDeclaration *decl, std::string &arg1str);
|
||
void DtoCheckPragma(PragmaDeclaration *decl, Dsymbol *sym,
|
||
Pragma llvm_internal, const std::string &arg1str);
|
||
|
||
#endif // PRAGMA_H
|