mirror of
https://github.com/dlang/dmd.git
synced 2025-04-25 20:50:41 +03:00
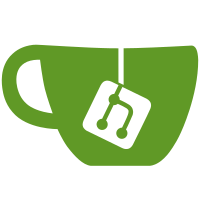
* Fix #21024 - Optimize x^^c expressions Reason for the *magic* constraint c<8 on inlining x^^c: https://sourceware.org/git/?p=glibc.git;a=blob;f=sysdeps/x86_64/fpu/e_powl.S;h=47f129f34d368d7c67b8e5f2462b36b0bebb7621;hb=HEAD#l136 * Fix poor assumption about expression state * Restrict optimization to floating point expressions * Generalize optimization to any scalar data type * Fix segfault on x^^c where x is a single member anonymous enum DMD segfaulted on compiling the unittests in std/algorithm/sorting.o, the unittest that caused the segfault can be reduced to: enum real Two = 2.0; auto _ = Two^^3; I'm not sure why copying the anonymous enum into a `const` variable causes the compiler to segfault. * Add tests to x^^c inlining optimization * Fix missing type for e1 ^^ -1 to 1 / e1 rewrite * Move rewrites from constant folding to expression semantic and restrict them to [-1, 2] * Improve error message for the x^^2 rewrite. Before: ex.d(4): Error: can implicitly convert expression `(const const(double) __powtmp2 = x + 5.0;) , __powtmp2 * ...` of type `double` to `int` int y = ( x + 5 ) ^^ 2; ^ and after: ex.d(4): Error: cannot implicitly convert expression `(x + 5.0) ^^ 2L` of type `double` to `int` int y = ( x + 5 ) ^^ 2; ^ * Update C++ frontend header to match change in `CommaExp` * Address code review feedback Co-authored-by: Dennis Korpel <dkorpel@gmail.com> --------- Co-authored-by: Dennis Korpel <dkorpel@gmail.com>
38 lines
801 B
D
38 lines
801 B
D
/*
|
|
TEST_OUTPUT:
|
|
---
|
|
fail_compilation/powinline.d(25): Error: cannot implicitly convert expression `(a + 5.0) ^^ 2L` of type `double` to `int`
|
|
fail_compilation/powinline.d(26): Error: cannot implicitly convert expression `(1.0 / foo()) ^^ 2L` of type `double` to `int`
|
|
fail_compilation/powinline.d(31): Error: void has no value
|
|
fail_compilation/powinline.d(31): Error: incompatible types for `(5.0) * (bar())`: `double` and `void`
|
|
fail_compilation/powinline.d(37): Error: cannot modify `immutable` expression `a`
|
|
---
|
|
*/
|
|
|
|
double foo()
|
|
{
|
|
return 5.0;
|
|
}
|
|
|
|
void bar()
|
|
{
|
|
return;
|
|
}
|
|
|
|
void test1()
|
|
{
|
|
double a = 2.0;
|
|
int b = (a + 5.0) ^^ 2.0;
|
|
b = (1 / foo()) ^^ 2.0;
|
|
}
|
|
|
|
void test2()
|
|
{
|
|
double a = (5.0 * bar()) ^^ 2.0;
|
|
}
|
|
|
|
void test3()
|
|
{
|
|
immutable double a = 3.0;
|
|
(a ^^= 2.0) = 6;
|
|
}
|